This tutorial will cover how to implement soft shadows in OpenGL 4.0 using GLSL and C++.
The code in this tutorial is based on the shadow mapping tutorial 41.
One of the issues with shadow maps is the lack of precision in the texture used for the shadow map.
Even high-resolution textures such as 1024x1024 (as was used in the shadow mapping tutorial) still create shadows with jagged edges.
For example, looking at the edge of the shadow for the sphere we get the following visual artifact:
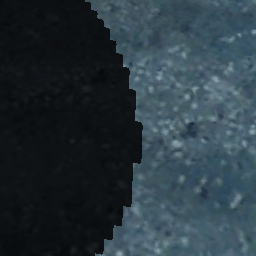
With that said one of the main advantages of using shadow maps is that we can easily produce soft shadows to fix the jagged shadow edges.
To create soft shadows, we first need to modify the shadow shader.
Instead of using the shader to render the shadowed scene with lighting as it previously did it will now instead just render shadow areas as pure black and the illuminated areas as pure white.
So, rendering our scene will now produce a black and white image as follows:
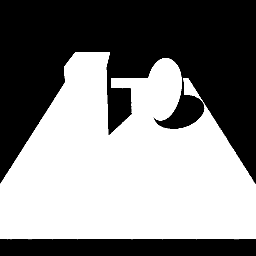
Also, we render the black and white scene to a render to texture instead of to the back buffer.
The reason we render to a texture is so that we can perform the next step which is to blur the black and white image.
For this tutorial we will perform a regular blur like we did in the blur tutorial (Tutorial 36).
With the black and white texture blurred we now have the following texture result:
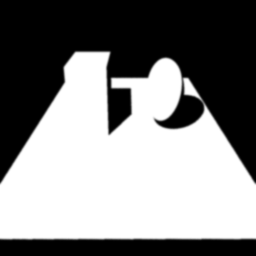
As you can see the shadow edges are now blurred creating the soft shadows.
To apply the soft shadows to the final scene we use just a regular lighting shader except that it takes as input the blurred image and at the end of the pixel shader we multiple the color result by the projected blur image to get soft shadows.
We project the blurred image from the camera's view point.
Doing so gives us the following soft shadow scene:
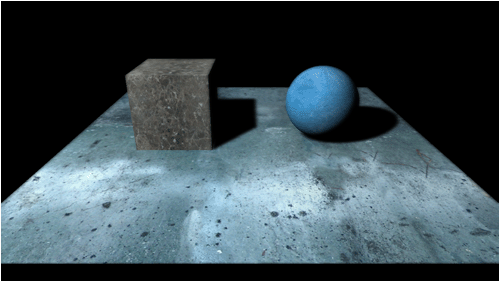
Framework
The new class we will be adding to our frame work is the SoftShadowShaderClass which will do the second step of soft shadow rendering.
To perform the blur of the black and white shadow render texture we will need to add our blurring related classes from the blur tutorial 36: BlurClass, BlurShaderClass, OrthoWindowClass, and TextureShaderClass.
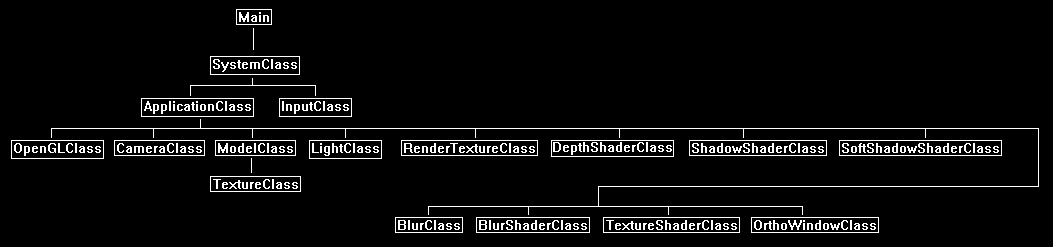
We will start the code section of the tutorial by looking at the modified shadow shader.
Shadow.vs
The vertex shader can remain the same.
////////////////////////////////////////////////////////////////////////////////
// Filename: shadow.vs
////////////////////////////////////////////////////////////////////////////////
#version 400
/////////////////////
// INPUT VARIABLES //
/////////////////////
in vec3 inputPosition;
in vec2 inputTexCoord;
in vec3 inputNormal;
//////////////////////
// OUTPUT VARIABLES //
//////////////////////
out vec2 texCoord;
out vec3 normal;
out vec4 lightViewPosition;
out vec3 lightPos;
///////////////////////
// UNIFORM VARIABLES //
///////////////////////
uniform mat4 worldMatrix;
uniform mat4 viewMatrix;
uniform mat4 projectionMatrix;
uniform mat4 lightViewMatrix;
uniform mat4 lightProjectionMatrix;
uniform vec3 lightPosition;
////////////////////////////////////////////////////////////////////////////////
// Vertex Shader
////////////////////////////////////////////////////////////////////////////////
void main(void)
{
vec4 worldPosition;
// Calculate the position of the vertex against the world, view, and projection matrices.
gl_Position = vec4(inputPosition, 1.0f) * worldMatrix;
gl_Position = gl_Position * viewMatrix;
gl_Position = gl_Position * projectionMatrix;
// Store the position of the vertice as viewed by the projection view point in a separate variable.
lightViewPosition = vec4(inputPosition, 1.0f) * worldMatrix;
lightViewPosition = lightViewPosition * lightViewMatrix;
lightViewPosition = lightViewPosition * lightProjectionMatrix;
// Store the texture coordinates for the pixel shader.
texCoord = inputTexCoord;
// Calculate the normal vector against the world matrix only.
normal = inputNormal * mat3(worldMatrix);
// Normalize the normal vector.
normal = normalize(normal);
// Calculate the position of the vertex in the world.
worldPosition = vec4(inputPosition, 1.0f) * worldMatrix;
// Determine the light position based on the position of the light and the position of the vertex in the world.
lightPos = lightPosition.xyz - worldPosition.xyz;
// Normalize the light position vector.
lightPos = normalize(lightPos);
}
Shadow.ps
In the pixel shader we have removed a number of the inputs since lighting and texturing are no longer needed to produce the pure black and white shadow image.
////////////////////////////////////////////////////////////////////////////////
// Filename: shadow.ps
////////////////////////////////////////////////////////////////////////////////
#version 400
/////////////////////
// INPUT VARIABLES //
/////////////////////
in vec2 texCoord;
in vec3 normal;
in vec4 lightViewPosition;
in vec3 lightPos;
//////////////////////
// OUTPUT VARIABLES //
//////////////////////
out vec4 outputColor;
///////////////////////
// UNIFORM VARIABLES //
///////////////////////
uniform sampler2D depthMapTexture;
uniform float bias;
////////////////////////////////////////////////////////////////////////////////
// Pixel Shader
////////////////////////////////////////////////////////////////////////////////
void main(void)
{
vec4 color;
vec2 projectTexCoord;
float depthValue;
float lightDepthValue;
float lightIntensity;
Now in the pixel shader we set the default color to be black (shadowed).
// Set the default output color to be black (shadow).
color = vec4(0.0f, 0.0f, 0.0f, 1.0f);
// Calculate the projected texture coordinates.
projectTexCoord.x = lightViewPosition.x / lightViewPosition.w / 2.0f + 0.5f;
projectTexCoord.y = lightViewPosition.y / lightViewPosition.w / 2.0f + 0.5f;
// Determine if the projected coordinates are in the 0 to 1 range. If so then this pixel is in the view of the light.
if((clamp(projectTexCoord.x, 0.0, 1.0) == projectTexCoord.x) && (clamp(projectTexCoord.y, 0.0, 1.0) == projectTexCoord.y))
{
// Sample the shadow map depth value from the depth texture using the sampler at the projected texture coordinate location.
depthValue = texture(depthMapTexture, projectTexCoord).r;
// Calculate the depth of the light.
lightDepthValue = lightViewPosition.z / lightViewPosition.w;
// Subtract the bias from the lightDepthValue.
lightDepthValue = lightDepthValue - bias;
// Compare the depth of the shadow map value and the depth of the light to determine whether to shadow or to light this pixel.
// If the light is in front of the object then light the pixel, if not then shadow this pixel since an object (occluder) is casting a shadow on it.
if(lightDepthValue < depthValue)
{
// Calculate the amount of light on this pixel.
lightIntensity = clamp(dot(normal, lightPos), 0.0f, 1.0f);
// If this is not in the shadow then set it's color to be white.
if(lightIntensity > 0.0f)
{
And if we determine a pixel is illuminated then it is colored pure white.
color = vec4(1.0f, 1.0f, 1.0f, 1.0f);
}
}
}
// Set the output color.
outputColor = color;
}
Shadowshaderclass.h
The ShadowShaderClass has had just the texturing and lighting color elements removed from it since it only needs to produce an un-textured black and white image.
Otherwise, it remains the same.
////////////////////////////////////////////////////////////////////////////////
// Filename: shadowshaderclass.h
////////////////////////////////////////////////////////////////////////////////
#ifndef _SHADOWSHADERCLASS_H_
#define _SHADOWSHADERCLASS_H_
//////////////
// INCLUDES //
//////////////
#include <iostream>
using namespace std;
///////////////////////
// MY CLASS INCLUDES //
///////////////////////
#include "openglclass.h"
////////////////////////////////////////////////////////////////////////////////
// Class name: ShadowShaderClass
////////////////////////////////////////////////////////////////////////////////
class ShadowShaderClass
{
public:
ShadowShaderClass();
ShadowShaderClass(const ShadowShaderClass&);
~ShadowShaderClass();
bool Initialize(OpenGLClass*);
void Shutdown();
bool SetShaderParameters(float*, float*, float*, float*, float*, float*, float);
private:
bool InitializeShader(char*, char*);
void ShutdownShader();
char* LoadShaderSourceFile(char*);
void OutputShaderErrorMessage(unsigned int, char*);
void OutputLinkerErrorMessage(unsigned int);
private:
OpenGLClass* m_OpenGLPtr;
unsigned int m_vertexShader;
unsigned int m_fragmentShader;
unsigned int m_shaderProgram;
};
#endif
Shadowshaderclass.cpp
////////////////////////////////////////////////////////////////////////////////
// Filename: shadowshaderclass.cpp
////////////////////////////////////////////////////////////////////////////////
#include "shadowshaderclass.h"
ShadowShaderClass::ShadowShaderClass()
{
m_OpenGLPtr = 0;
}
ShadowShaderClass::ShadowShaderClass(const ShadowShaderClass& other)
{
}
ShadowShaderClass::~ShadowShaderClass()
{
}
bool ShadowShaderClass::Initialize(OpenGLClass* OpenGL)
{
char vsFilename[128];
char psFilename[128];
bool result;
// Store the pointer to the OpenGL object.
m_OpenGLPtr = OpenGL;
// Set the location and names of the shader files.
strcpy(vsFilename, "../Engine/shadow.vs");
strcpy(psFilename, "../Engine/shadow.ps");
// Initialize the vertex and pixel shaders.
result = InitializeShader(vsFilename, psFilename);
if(!result)
{
return false;
}
return true;
}
void ShadowShaderClass::Shutdown()
{
// Shutdown the shader.
ShutdownShader();
// Release the pointer to the OpenGL object.
m_OpenGLPtr = 0;
return;
}
bool ShadowShaderClass::InitializeShader(char* vsFilename, char* fsFilename)
{
const char* vertexShaderBuffer;
const char* fragmentShaderBuffer;
int status;
// Load the vertex shader source file into a text buffer.
vertexShaderBuffer = LoadShaderSourceFile(vsFilename);
if(!vertexShaderBuffer)
{
return false;
}
// Load the fragment shader source file into a text buffer.
fragmentShaderBuffer = LoadShaderSourceFile(fsFilename);
if(!fragmentShaderBuffer)
{
return false;
}
// Create a vertex and fragment shader object.
m_vertexShader = m_OpenGLPtr->glCreateShader(GL_VERTEX_SHADER);
m_fragmentShader = m_OpenGLPtr->glCreateShader(GL_FRAGMENT_SHADER);
// Copy the shader source code strings into the vertex and fragment shader objects.
m_OpenGLPtr->glShaderSource(m_vertexShader, 1, &vertexShaderBuffer, NULL);
m_OpenGLPtr->glShaderSource(m_fragmentShader, 1, &fragmentShaderBuffer, NULL);
// Release the vertex and fragment shader buffers.
delete [] vertexShaderBuffer;
vertexShaderBuffer = 0;
delete [] fragmentShaderBuffer;
fragmentShaderBuffer = 0;
// Compile the shaders.
m_OpenGLPtr->glCompileShader(m_vertexShader);
m_OpenGLPtr->glCompileShader(m_fragmentShader);
// Check to see if the vertex shader compiled successfully.
m_OpenGLPtr->glGetShaderiv(m_vertexShader, GL_COMPILE_STATUS, &status);
if(status != 1)
{
// If it did not compile then write the syntax error message out to a text file for review.
OutputShaderErrorMessage(m_vertexShader, vsFilename);
return false;
}
// Check to see if the fragment shader compiled successfully.
m_OpenGLPtr->glGetShaderiv(m_fragmentShader, GL_COMPILE_STATUS, &status);
if(status != 1)
{
// If it did not compile then write the syntax error message out to a text file for review.
OutputShaderErrorMessage(m_fragmentShader, fsFilename);
return false;
}
// Create a shader program object.
m_shaderProgram = m_OpenGLPtr->glCreateProgram();
// Attach the vertex and fragment shader to the program object.
m_OpenGLPtr->glAttachShader(m_shaderProgram, m_vertexShader);
m_OpenGLPtr->glAttachShader(m_shaderProgram, m_fragmentShader);
// Bind the shader input variables.
m_OpenGLPtr->glBindAttribLocation(m_shaderProgram, 0, "inputPosition");
m_OpenGLPtr->glBindAttribLocation(m_shaderProgram, 1, "inputTexCoord");
m_OpenGLPtr->glBindAttribLocation(m_shaderProgram, 2, "inputNormal");
// Link the shader program.
m_OpenGLPtr->glLinkProgram(m_shaderProgram);
// Check the status of the link.
m_OpenGLPtr->glGetProgramiv(m_shaderProgram, GL_LINK_STATUS, &status);
if(status != 1)
{
// If it did not link then write the syntax error message out to a text file for review.
OutputLinkerErrorMessage(m_shaderProgram);
return false;
}
return true;
}
void ShadowShaderClass::ShutdownShader()
{
// Detach the vertex and fragment shaders from the program.
m_OpenGLPtr->glDetachShader(m_shaderProgram, m_vertexShader);
m_OpenGLPtr->glDetachShader(m_shaderProgram, m_fragmentShader);
// Delete the vertex and fragment shaders.
m_OpenGLPtr->glDeleteShader(m_vertexShader);
m_OpenGLPtr->glDeleteShader(m_fragmentShader);
// Delete the shader program.
m_OpenGLPtr->glDeleteProgram(m_shaderProgram);
return;
}
char* ShadowShaderClass::LoadShaderSourceFile(char* filename)
{
FILE* filePtr;
char* buffer;
long fileSize, count;
int error;
// Open the shader file for reading in text modee.
filePtr = fopen(filename, "r");
if(filePtr == NULL)
{
return 0;
}
// Go to the end of the file and get the size of the file.
fseek(filePtr, 0, SEEK_END);
fileSize = ftell(filePtr);
// Initialize the buffer to read the shader source file into, adding 1 for an extra null terminator.
buffer = new char[fileSize + 1];
// Return the file pointer back to the beginning of the file.
fseek(filePtr, 0, SEEK_SET);
// Read the shader text file into the buffer.
count = fread(buffer, 1, fileSize, filePtr);
if(count != fileSize)
{
return 0;
}
// Close the file.
error = fclose(filePtr);
if(error != 0)
{
return 0;
}
// Null terminate the buffer.
buffer[fileSize] = '\0';
return buffer;
}
void ShadowShaderClass::OutputShaderErrorMessage(unsigned int shaderId, char* shaderFilename)
{
long count;
int logSize, error;
char* infoLog;
FILE* filePtr;
// Get the size of the string containing the information log for the failed shader compilation message.
m_OpenGLPtr->glGetShaderiv(shaderId, GL_INFO_LOG_LENGTH, &logSize);
// Increment the size by one to handle also the null terminator.
logSize++;
// Create a char buffer to hold the info log.
infoLog = new char[logSize];
// Now retrieve the info log.
m_OpenGLPtr->glGetShaderInfoLog(shaderId, logSize, NULL, infoLog);
// Open a text file to write the error message to.
filePtr = fopen("shader-error.txt", "w");
if(filePtr == NULL)
{
cout << "Error opening shader error message output file." << endl;
return;
}
// Write out the error message.
count = fwrite(infoLog, sizeof(char), logSize, filePtr);
if(count != logSize)
{
cout << "Error writing shader error message output file." << endl;
return;
}
// Close the file.
error = fclose(filePtr);
if(error != 0)
{
cout << "Error closing shader error message output file." << endl;
return;
}
// Notify the user to check the text file for compile errors.
cout << "Error compiling shader. Check shader-error.txt for error message. Shader filename: " << shaderFilename << endl;
return;
}
void ShadowShaderClass::OutputLinkerErrorMessage(unsigned int programId)
{
long count;
FILE* filePtr;
int logSize, error;
char* infoLog;
// Get the size of the string containing the information log for the failed shader compilation message.
m_OpenGLPtr->glGetProgramiv(programId, GL_INFO_LOG_LENGTH, &logSize);
// Increment the size by one to handle also the null terminator.
logSize++;
// Create a char buffer to hold the info log.
infoLog = new char[logSize];
// Now retrieve the info log.
m_OpenGLPtr->glGetProgramInfoLog(programId, logSize, NULL, infoLog);
// Open a file to write the error message to.
filePtr = fopen("linker-error.txt", "w");
if(filePtr == NULL)
{
cout << "Error opening linker error message output file." << endl;
return;
}
// Write out the error message.
count = fwrite(infoLog, sizeof(char), logSize, filePtr);
if(count != logSize)
{
cout << "Error writing linker error message output file." << endl;
return;
}
// Close the file.
error = fclose(filePtr);
if(error != 0)
{
cout << "Error closing linker error message output file." << endl;
return;
}
// Pop a message up on the screen to notify the user to check the text file for linker errors.
cout << "Error linking shader program. Check linker-error.txt for message." << endl;
return;
}
The SetShaderParameters function has had some of the variables removed that we won't need for producing just a black and white version of the scene.
bool ShadowShaderClass::SetShaderParameters(float* worldMatrix, float* viewMatrix, float* projectionMatrix, float* lightViewMatrix, float* lightProjectionMatrix,
float* lightPosition, float bias)
{
float tpWorldMatrix[16], tpViewMatrix[16], tpProjectionMatrix[16], tpLightViewMatrix[16], tpLightProjectionMatrix[16];
int location;
// Transpose the matrices to prepare them for the shader.
m_OpenGLPtr->MatrixTranspose(tpWorldMatrix, worldMatrix);
m_OpenGLPtr->MatrixTranspose(tpViewMatrix, viewMatrix);
m_OpenGLPtr->MatrixTranspose(tpProjectionMatrix, projectionMatrix);
// Transpose the second view and projection matrices.
m_OpenGLPtr->MatrixTranspose(tpLightViewMatrix, lightViewMatrix);
m_OpenGLPtr->MatrixTranspose(tpLightProjectionMatrix, lightProjectionMatrix);
// Install the shader program as part of the current rendering state.
m_OpenGLPtr->glUseProgram(m_shaderProgram);
// Set the world matrix in the vertex shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "worldMatrix");
if(location == -1)
{
cout << "World matrix not set." << endl;
}
m_OpenGLPtr->glUniformMatrix4fv(location, 1, false, tpWorldMatrix);
// Set the view matrix in the vertex shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "viewMatrix");
if(location == -1)
{
cout << "View matrix not set." << endl;
}
m_OpenGLPtr->glUniformMatrix4fv(location, 1, false, tpViewMatrix);
// Set the projection matrix in the vertex shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "projectionMatrix");
if(location == -1)
{
cout << "Projection matrix not set." << endl;
}
m_OpenGLPtr->glUniformMatrix4fv(location, 1, false, tpProjectionMatrix);
// Set the light view matrix in the vertex shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "lightViewMatrix");
if(location == -1)
{
cout << "Light view matrix not set." << endl;
}
m_OpenGLPtr->glUniformMatrix4fv(location, 1, false, tpLightViewMatrix);
// Set the light projection matrix in the vertex shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "lightProjectionMatrix");
if(location == -1)
{
cout << "Light projection matrix not set." << endl;
}
m_OpenGLPtr->glUniformMatrix4fv(location, 1, false, tpLightProjectionMatrix);
// Set the light position in the pixel shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "lightPosition");
if(location == -1)
{
cout << "Light position not set." << endl;
}
m_OpenGLPtr->glUniform3fv(location, 1, lightPosition);
// Set the projection texture in the pixel shader to use the data from the first texture unit.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "depthMapTexture");
if(location == -1)
{
cout << "Depth map texture not set." << endl;
}
m_OpenGLPtr->glUniform1i(location, 0);
// Set the shadow map bias in the pixel shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "bias");
if(location == -1)
{
cout << "Bias not set." << endl;
}
m_OpenGLPtr->glUniform1f(location, bias);
return true;
}
Softshadow.vs
The soft shadow shaders are where we light the scene as normal but then project the blurred black and white shadow texture onto the scene to complete the soft shadow effect.
The vertex shader is mostly similar to the original shadow vertex shader with some slight changes.
////////////////////////////////////////////////////////////////////////////////
// Filename: softshadow.vs
////////////////////////////////////////////////////////////////////////////////
#version 400
/////////////////////
// INPUT VARIABLES //
/////////////////////
in vec3 inputPosition;
in vec2 inputTexCoord;
in vec3 inputNormal;
//////////////////////
// OUTPUT VARIABLES //
//////////////////////
out vec2 texCoord;
out vec3 normal;
out vec4 viewPosition;
out vec3 lightPos;
///////////////////////
// UNIFORM VARIABLES //
///////////////////////
uniform mat4 worldMatrix;
uniform mat4 viewMatrix;
uniform mat4 projectionMatrix;
uniform vec3 lightPosition;
////////////////////////////////////////////////////////////////////////////////
// Vertex Shader
////////////////////////////////////////////////////////////////////////////////
void main(void)
{
vec4 worldPosition;
// Calculate the position of the vertex against the world, view, and projection matrices.
gl_Position = vec4(inputPosition, 1.0f) * worldMatrix;
gl_Position = gl_Position * viewMatrix;
gl_Position = gl_Position * projectionMatrix;
The viewPosition will be used to calculate the projection coordinates to project the soft shadows onto the scene.
// Store the position of the vertice as viewed by the camera in a separate variable.
viewPosition = gl_Position;
// Store the texture coordinates for the pixel shader.
texCoord = inputTexCoord;
// Calculate the normal vector against the world matrix only.
normal = inputNormal * mat3(worldMatrix);
// Normalize the normal vector.
normal = normalize(normal);
// Calculate the position of the vertex in the world.
worldPosition = vec4(inputPosition, 1.0f) * worldMatrix;
// Determine the light position based on the position of the light and the position of the vertex in the world.
lightPos = lightPosition.xyz - worldPosition.xyz;
// Normalize the light position vector.
lightPos = normalize(lightPos);
}
Softshadow.ps
////////////////////////////////////////////////////////////////////////////////
// Filename: softshadow.ps
////////////////////////////////////////////////////////////////////////////////
#version 400
/////////////////////
// INPUT VARIABLES //
/////////////////////
in vec2 texCoord;
in vec3 normal;
in vec4 viewPosition;
in vec3 lightPos;
//////////////////////
// OUTPUT VARIABLES //
//////////////////////
out vec4 outputColor;
///////////////////////
// UNIFORM VARIABLES //
///////////////////////
uniform sampler2D shaderTexture;
The shadowTexture is the black and white blurred image that contains the soft shadows.
We will project this texture onto the scene.
uniform sampler2D shadowTexture;
uniform vec4 ambientColor;
uniform vec4 diffuseColor;
////////////////////////////////////////////////////////////////////////////////
// Pixel Shader
////////////////////////////////////////////////////////////////////////////////
void main(void)
{
vec4 color;
float lightIntensity;
vec4 textureColor;
vec2 projectTexCoord;
float shadowValue;
Calculate the lighting and texturing as normal.
// Set the default output color to the ambient light value for all pixels.
color = ambientColor;
// Calculate the amount of light on this pixel.
lightIntensity = clamp(dot(normal, lightPos), 0.0f, 1.0f);
if(lightIntensity > 0.0f)
{
// Determine the final diffuse color based on the diffuse color and the amount of light intensity.
color += (diffuseColor * lightIntensity);
// Saturate the light color.
color = clamp(color, 0.0f, 1.0f);
}
// Sample the pixel color from the texture using the sampler at this texture coordinate location.
textureColor = texture(shaderTexture, texCoord);
// Combine the light and texture color.
color = color * textureColor;
Now calculate the projected coordinates to sample the blurred black and white image from based on the camera's view point.
// Calculate the projected texture coordinates to be used with the shadow texture.
projectTexCoord.x = viewPosition.x / viewPosition.w / 2.0f + 0.5f;
projectTexCoord.y = viewPosition.y / viewPosition.w / 2.0f + 0.5f;
Sample the soft shadow image using the projected coordinates and then multiple it in the same fashion as a light map to the final color to get the soft shadow effect.
// Sample the shadow map depth value from the depth texture using the sampler at the projected texture coordinate location.
shadowValue = texture(shadowTexture, projectTexCoord).r;
// Combine the shadows with the final color.
outputColor = color * shadowValue;
}
Softshadowshaderclass.h
////////////////////////////////////////////////////////////////////////////////
// Filename: softshadowshaderclass.h
////////////////////////////////////////////////////////////////////////////////
#ifndef _SOFTSHADOWSHADERCLASS_H_
#define _SOFTSHADOWSHADERCLASS_H_
//////////////
// INCLUDES //
//////////////
#include <iostream>
using namespace std;
///////////////////////
// MY CLASS INCLUDES //
///////////////////////
#include "openglclass.h"
////////////////////////////////////////////////////////////////////////////////
// Class name: SoftShadowShaderClass
////////////////////////////////////////////////////////////////////////////////
class SoftShadowShaderClass
{
public:
SoftShadowShaderClass();
SoftShadowShaderClass(const SoftShadowShaderClass&);
~SoftShadowShaderClass();
bool Initialize(OpenGLClass*);
void Shutdown();
bool SetShaderParameters(float*, float*, float*, float*, float*, float*);
private:
bool InitializeShader(char*, char*);
void ShutdownShader();
char* LoadShaderSourceFile(char*);
void OutputShaderErrorMessage(unsigned int, char*);
void OutputLinkerErrorMessage(unsigned int);
private:
OpenGLClass* m_OpenGLPtr;
unsigned int m_vertexShader;
unsigned int m_fragmentShader;
unsigned int m_shaderProgram;
};
#endif
Softshadowshaderclass.cpp
////////////////////////////////////////////////////////////////////////////////
// Filename: softshadowshaderclass.cpp
////////////////////////////////////////////////////////////////////////////////
#include "softshadowshaderclass.h"
SoftShadowShaderClass::SoftShadowShaderClass()
{
m_OpenGLPtr = 0;
}
SoftShadowShaderClass::SoftShadowShaderClass(const SoftShadowShaderClass& other)
{
}
SoftShadowShaderClass::~SoftShadowShaderClass()
{
}
bool SoftShadowShaderClass::Initialize(OpenGLClass* OpenGL)
{
char vsFilename[128];
char psFilename[128];
bool result;
// Store the pointer to the OpenGL object.
m_OpenGLPtr = OpenGL;
// Set the location and names of the shader files.
strcpy(vsFilename, "../Engine/softshadow.vs");
strcpy(psFilename, "../Engine/softshadow.ps");
// Initialize the vertex and pixel shaders.
result = InitializeShader(vsFilename, psFilename);
if(!result)
{
return false;
}
return true;
}
void SoftShadowShaderClass::Shutdown()
{
// Shutdown the shader.
ShutdownShader();
// Release the pointer to the OpenGL object.
m_OpenGLPtr = 0;
return;
}
bool SoftShadowShaderClass::InitializeShader(char* vsFilename, char* fsFilename)
{
const char* vertexShaderBuffer;
const char* fragmentShaderBuffer;
int status;
// Load the vertex shader source file into a text buffer.
vertexShaderBuffer = LoadShaderSourceFile(vsFilename);
if(!vertexShaderBuffer)
{
return false;
}
// Load the fragment shader source file into a text buffer.
fragmentShaderBuffer = LoadShaderSourceFile(fsFilename);
if(!fragmentShaderBuffer)
{
return false;
}
// Create a vertex and fragment shader object.
m_vertexShader = m_OpenGLPtr->glCreateShader(GL_VERTEX_SHADER);
m_fragmentShader = m_OpenGLPtr->glCreateShader(GL_FRAGMENT_SHADER);
// Copy the shader source code strings into the vertex and fragment shader objects.
m_OpenGLPtr->glShaderSource(m_vertexShader, 1, &vertexShaderBuffer, NULL);
m_OpenGLPtr->glShaderSource(m_fragmentShader, 1, &fragmentShaderBuffer, NULL);
// Release the vertex and fragment shader buffers.
delete [] vertexShaderBuffer;
vertexShaderBuffer = 0;
delete [] fragmentShaderBuffer;
fragmentShaderBuffer = 0;
// Compile the shaders.
m_OpenGLPtr->glCompileShader(m_vertexShader);
m_OpenGLPtr->glCompileShader(m_fragmentShader);
// Check to see if the vertex shader compiled successfully.
m_OpenGLPtr->glGetShaderiv(m_vertexShader, GL_COMPILE_STATUS, &status);
if(status != 1)
{
// If it did not compile then write the syntax error message out to a text file for review.
OutputShaderErrorMessage(m_vertexShader, vsFilename);
return false;
}
// Check to see if the fragment shader compiled successfully.
m_OpenGLPtr->glGetShaderiv(m_fragmentShader, GL_COMPILE_STATUS, &status);
if(status != 1)
{
// If it did not compile then write the syntax error message out to a text file for review.
OutputShaderErrorMessage(m_fragmentShader, fsFilename);
return false;
}
// Create a shader program object.
m_shaderProgram = m_OpenGLPtr->glCreateProgram();
// Attach the vertex and fragment shader to the program object.
m_OpenGLPtr->glAttachShader(m_shaderProgram, m_vertexShader);
m_OpenGLPtr->glAttachShader(m_shaderProgram, m_fragmentShader);
// Bind the shader input variables.
m_OpenGLPtr->glBindAttribLocation(m_shaderProgram, 0, "inputPosition");
m_OpenGLPtr->glBindAttribLocation(m_shaderProgram, 1, "inputTexCoord");
m_OpenGLPtr->glBindAttribLocation(m_shaderProgram, 2, "inputNormal");
// Link the shader program.
m_OpenGLPtr->glLinkProgram(m_shaderProgram);
// Check the status of the link.
m_OpenGLPtr->glGetProgramiv(m_shaderProgram, GL_LINK_STATUS, &status);
if(status != 1)
{
// If it did not link then write the syntax error message out to a text file for review.
OutputLinkerErrorMessage(m_shaderProgram);
return false;
}
return true;
}
void SoftShadowShaderClass::ShutdownShader()
{
// Detach the vertex and fragment shaders from the program.
m_OpenGLPtr->glDetachShader(m_shaderProgram, m_vertexShader);
m_OpenGLPtr->glDetachShader(m_shaderProgram, m_fragmentShader);
// Delete the vertex and fragment shaders.
m_OpenGLPtr->glDeleteShader(m_vertexShader);
m_OpenGLPtr->glDeleteShader(m_fragmentShader);
// Delete the shader program.
m_OpenGLPtr->glDeleteProgram(m_shaderProgram);
return;
}
char* SoftShadowShaderClass::LoadShaderSourceFile(char* filename)
{
FILE* filePtr;
char* buffer;
long fileSize, count;
int error;
// Open the shader file for reading in text modee.
filePtr = fopen(filename, "r");
if(filePtr == NULL)
{
return 0;
}
// Go to the end of the file and get the size of the file.
fseek(filePtr, 0, SEEK_END);
fileSize = ftell(filePtr);
// Initialize the buffer to read the shader source file into, adding 1 for an extra null terminator.
buffer = new char[fileSize + 1];
// Return the file pointer back to the beginning of the file.
fseek(filePtr, 0, SEEK_SET);
// Read the shader text file into the buffer.
count = fread(buffer, 1, fileSize, filePtr);
if(count != fileSize)
{
return 0;
}
// Close the file.
error = fclose(filePtr);
if(error != 0)
{
return 0;
}
// Null terminate the buffer.
buffer[fileSize] = '\0';
return buffer;
}
void SoftShadowShaderClass::OutputShaderErrorMessage(unsigned int shaderId, char* shaderFilename)
{
long count;
int logSize, error;
char* infoLog;
FILE* filePtr;
// Get the size of the string containing the information log for the failed shader compilation message.
m_OpenGLPtr->glGetShaderiv(shaderId, GL_INFO_LOG_LENGTH, &logSize);
// Increment the size by one to handle also the null terminator.
logSize++;
// Create a char buffer to hold the info log.
infoLog = new char[logSize];
// Now retrieve the info log.
m_OpenGLPtr->glGetShaderInfoLog(shaderId, logSize, NULL, infoLog);
// Open a text file to write the error message to.
filePtr = fopen("shader-error.txt", "w");
if(filePtr == NULL)
{
cout << "Error opening shader error message output file." << endl;
return;
}
// Write out the error message.
count = fwrite(infoLog, sizeof(char), logSize, filePtr);
if(count != logSize)
{
cout << "Error writing shader error message output file." << endl;
return;
}
// Close the file.
error = fclose(filePtr);
if(error != 0)
{
cout << "Error closing shader error message output file." << endl;
return;
}
// Notify the user to check the text file for compile errors.
cout << "Error compiling shader. Check shader-error.txt for error message. Shader filename: " << shaderFilename << endl;
return;
}
void SoftShadowShaderClass::OutputLinkerErrorMessage(unsigned int programId)
{
long count;
FILE* filePtr;
int logSize, error;
char* infoLog;
// Get the size of the string containing the information log for the failed shader compilation message.
m_OpenGLPtr->glGetProgramiv(programId, GL_INFO_LOG_LENGTH, &logSize);
// Increment the size by one to handle also the null terminator.
logSize++;
// Create a char buffer to hold the info log.
infoLog = new char[logSize];
// Now retrieve the info log.
m_OpenGLPtr->glGetProgramInfoLog(programId, logSize, NULL, infoLog);
// Open a file to write the error message to.
filePtr = fopen("linker-error.txt", "w");
if(filePtr == NULL)
{
cout << "Error opening linker error message output file." << endl;
return;
}
// Write out the error message.
count = fwrite(infoLog, sizeof(char), logSize, filePtr);
if(count != logSize)
{
cout << "Error writing linker error message output file." << endl;
return;
}
// Close the file.
error = fclose(filePtr);
if(error != 0)
{
cout << "Error closing linker error message output file." << endl;
return;
}
// Pop a message up on the screen to notify the user to check the text file for linker errors.
cout << "Error linking shader program. Check linker-error.txt for message." << endl;
return;
}
bool SoftShadowShaderClass::SetShaderParameters(float* worldMatrix, float* viewMatrix, float* projectionMatrix, float* lightPosition, float* ambientColor, float* diffuseColor)
{
float tpWorldMatrix[16], tpViewMatrix[16], tpProjectionMatrix[16];
int location;
// Transpose the matrices to prepare them for the shader.
m_OpenGLPtr->MatrixTranspose(tpWorldMatrix, worldMatrix);
m_OpenGLPtr->MatrixTranspose(tpViewMatrix, viewMatrix);
m_OpenGLPtr->MatrixTranspose(tpProjectionMatrix, projectionMatrix);
// Install the shader program as part of the current rendering state.
m_OpenGLPtr->glUseProgram(m_shaderProgram);
// Set the world matrix in the vertex shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "worldMatrix");
if(location == -1)
{
cout << "World matrix not set." << endl;
}
m_OpenGLPtr->glUniformMatrix4fv(location, 1, false, tpWorldMatrix);
// Set the view matrix in the vertex shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "viewMatrix");
if(location == -1)
{
cout << "View matrix not set." << endl;
}
m_OpenGLPtr->glUniformMatrix4fv(location, 1, false, tpViewMatrix);
// Set the projection matrix in the vertex shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "projectionMatrix");
if(location == -1)
{
cout << "Projection matrix not set." << endl;
}
m_OpenGLPtr->glUniformMatrix4fv(location, 1, false, tpProjectionMatrix);
// Set the light position in the vertex shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "lightPosition");
if(location == -1)
{
cout << "Light position not set." << endl;
}
m_OpenGLPtr->glUniform3fv(location, 1, lightPosition);
// Set the texture in the pixel shader to use the data from the first texture unit.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "shaderTexture");
if(location == -1)
{
cout << "Shader texture not set." << endl;
}
m_OpenGLPtr->glUniform1i(location, 0);
The SetShaderParameters function is mostly the same as just a regular light shader.
However, the one addition is that we use the blurred black and white soft shadow texture in the second texture unit for projecting it onto the scene for soft shadow calculations.
// Set the shadow texture in the pixel shader to use the data from the second texture unit.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "shadowTexture");
if(location == -1)
{
cout << "Shadow texture not set." << endl;
}
m_OpenGLPtr->glUniform1i(location, 1);
// Set the ambient light in the pixel shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "ambientColor");
if(location == -1)
{
cout << "Ambient color not set." << endl;
}
m_OpenGLPtr->glUniform4fv(location, 1, ambientColor);
// Set the diffuse light color in the pixel shader.
location = m_OpenGLPtr->glGetUniformLocation(m_shaderProgram, "diffuseColor");
if(location == -1)
{
cout << "Diffuse color not set." << endl;
}
m_OpenGLPtr->glUniform4fv(location, 1, diffuseColor);
return true;
}
Applicationclass.h
////////////////////////////////////////////////////////////////////////////////
// Filename: applicationclass.h
////////////////////////////////////////////////////////////////////////////////
#ifndef _APPLICATIONCLASS_H_
#define _APPLICATIONCLASS_H_
/////////////
// GLOBALS //
/////////////
const bool FULL_SCREEN = false;
const bool VSYNC_ENABLED = true;
const float SCREEN_NEAR = 1.0f;
const float SCREEN_DEPTH = 100.0f;
const int SHADOWMAP_WIDTH = 1024;
const int SHADOWMAP_HEIGHT = 1024;
///////////////////////
// MY CLASS INCLUDES //
///////////////////////
#include "inputclass.h"
#include "openglclass.h"
#include "cameraclass.h"
#include "modelclass.h"
#include "lightclass.h"
#include "rendertextureclass.h"
#include "depthshaderclass.h"
#include "shadowshaderclass.h"
We have added the headers required for soft shadowing and blurring.
#include "softshadowshaderclass.h"
#include "blurclass.h"
////////////////////////////////////////////////////////////////////////////////
// Class Name: ApplicationClass
////////////////////////////////////////////////////////////////////////////////
class ApplicationClass
{
public:
ApplicationClass();
ApplicationClass(const ApplicationClass&);
~ApplicationClass();
bool Initialize(Display*, Window, int, int);
void Shutdown();
bool Frame(InputClass*);
private:
bool RenderDepthToTexture();
There is a new function for doing the black and white render pass on the scene.
bool RenderBlackAndWhiteShadows();
bool Render();
private:
OpenGLClass* m_OpenGL;
CameraClass* m_Camera;
ModelClass *m_CubeModel, *m_SphereModel, *m_GroundModel;
LightClass* m_Light;
We have added an additional render texture object for the black and white scene render.
RenderTextureClass *m_RenderTexture, *m_BlackWhiteRenderTexture;
DepthShaderClass* m_DepthShader;
ShadowShaderClass* m_ShadowShader;
The new SoftShadowShaderClass object is defined here.
SoftShadowShaderClass* m_SoftShadowShader;
The blurring objects have been added here so we can blur the black and white scene to produce soft shadows.
BlurClass* m_Blur;
TextureShaderClass* m_TextureShader;
BlurShaderClass* m_BlurShader;
float m_shadowMapBias;
};
#endif
Applicationclass.cpp
////////////////////////////////////////////////////////////////////////////////
// Filename: applicationclass.cpp
////////////////////////////////////////////////////////////////////////////////
#include "applicationclass.h"
Initialize the new class objects to null in the class constructor.
ApplicationClass::ApplicationClass()
{
m_OpenGL = 0;
m_Camera = 0;
m_CubeModel = 0;
m_SphereModel = 0;
m_GroundModel = 0;
m_Light = 0;
m_RenderTexture = 0;
m_BlackWhiteRenderTexture = 0;
m_DepthShader = 0;
m_ShadowShader = 0;
m_SoftShadowShader = 0;
m_Blur = 0;
m_TextureShader = 0;
m_BlurShader = 0;
}
ApplicationClass::ApplicationClass(const ApplicationClass& other)
{
}
ApplicationClass::~ApplicationClass()
{
}
bool ApplicationClass::Initialize(Display* display, Window win, int screenWidth, int screenHeight)
{
char modelFilename[128], textureFilename[128];
int downSampleWidth, downSampleHeight;
bool result;
// Create and initialize the OpenGL object.
m_OpenGL = new OpenGLClass;
result = m_OpenGL->Initialize(display, win, screenWidth, screenHeight, SCREEN_NEAR, SCREEN_DEPTH, VSYNC_ENABLED);
if(!result)
{
cout << "Error: Could not initialize the OpenGL object." << endl;
return false;
}
// Create and initialize the camera object.
m_Camera = new CameraClass;
We will need a base view matrix for the blur since the regular camera will be moved.
m_Camera->SetPosition(0.0f, 0.0f, -10.0f);
m_Camera->Render();
m_Camera->RenderBaseViewMatrix();
// Set the camera up a bit to view the shadows better.
m_Camera->SetPosition(0.0f, 7.0f, -10.0f);
m_Camera->SetRotation(35.0f, 0.0f, 0.0f);
m_Camera->Render();
// Create and initialize the cube model object.
m_CubeModel = new ModelClass;
strcpy(modelFilename, "../Engine/data/cube.txt");
strcpy(textureFilename, "../Engine/data/wall01.tga");
result = m_CubeModel->Initialize(m_OpenGL, modelFilename, textureFilename, false, NULL, false, NULL, false);
if(!result)
{
cout << "Error: Could not initialize the cube model object." << endl;
return false;
}
// Create and initialize the sphere model object.
m_SphereModel = new ModelClass;
strcpy(modelFilename, "../Engine/data/sphere.txt");
strcpy(textureFilename, "../Engine/data/ice.tga");
result = m_SphereModel->Initialize(m_OpenGL, modelFilename, textureFilename, true, NULL, false, NULL, false);
if(!result)
{
cout << "Error: Could not initialize the sphere model object." << endl;
return false;
}
// Create and initialize the ground model object.
m_GroundModel = new ModelClass;
strcpy(modelFilename, "../Engine/data/plane01.txt");
strcpy(textureFilename, "../Engine/data/metal001.tga");
result = m_GroundModel->Initialize(m_OpenGL, modelFilename, textureFilename, false, NULL, false, NULL, false);
if(!result)
{
cout << "Error: Could not initialize the ground model object." << endl;
return false;
}
// Create and initialize the light object.
m_Light = new LightClass;
m_Light->SetAmbientLight(0.15f, 0.15f, 0.15f, 1.0f);
m_Light->SetDiffuseColor(1.0f, 1.0f, 1.0f, 1.0f);
m_Light->SetLookAt(0.0f, 0.0f, 0.0f);
m_Light->GenerateProjectionMatrix(SCREEN_DEPTH, SCREEN_NEAR);
// Create and initialize the render to texture object.
m_RenderTexture = new RenderTextureClass;
result = m_RenderTexture->Initialize(m_OpenGL, SHADOWMAP_WIDTH, SHADOWMAP_HEIGHT, SCREEN_NEAR, SCREEN_DEPTH, 0);
if(!result)
{
cout << "Error: Could not initialize the render texture object." << endl;
return false;
}
This is where we initialize the new black and white render texture.
Note that we set its dimensions to that of the shadow map width and height globals.
// Create and initialize the black and white render to texture object.
m_BlackWhiteRenderTexture = new RenderTextureClass;
result = m_BlackWhiteRenderTexture->Initialize(m_OpenGL, SHADOWMAP_WIDTH, SHADOWMAP_HEIGHT, SCREEN_NEAR, SCREEN_DEPTH, 0);
if(!result)
{
cout << "Error: Could not initialize the render texture object." << endl;
return false;
}
// Create and initialize the depth shader object.
m_DepthShader = new DepthShaderClass;
result = m_DepthShader->Initialize(m_OpenGL);
if(!result)
{
cout << "Error: Could not initialize the depth shader object." << endl;
return false;
}
// Create and initialize the shadow shader object.
m_ShadowShader = new ShadowShaderClass;
result = m_ShadowShader->Initialize(m_OpenGL);
if(!result)
{
cout << "Error: Could not initialize the shadow shader object." << endl;
return false;
}
Here we initialize our new soft shadow shader.
// Create and initialize the soft shadow shader object.
m_SoftShadowShader = new SoftShadowShaderClass;
result = m_SoftShadowShader->Initialize(m_OpenGL);
if(!result)
{
cout << "Error: Could not initialize the soft shadow shader object." << endl;
return false;
}
Since we will be blurring the black and white render texture, and because the size of the texture was set to the shadow map sizes, then
we need to set our down sample size to use half that render texture size.
// Set the size to sample down to.
downSampleWidth = SHADOWMAP_WIDTH / 2;
downSampleHeight = SHADOWMAP_HEIGHT / 2;
The blur object will be created using the shadow map sizing for dimensions and down sample sizing.
// Create and initialize the blur object.
m_Blur = new BlurClass;
result = m_Blur->Initialize(m_OpenGL, downSampleWidth, downSampleHeight, SCREEN_NEAR, SCREEN_DEPTH, SHADOWMAP_WIDTH, SHADOWMAP_HEIGHT);
if(!result)
{
cout << "Error: Could not initialize the blur object." << endl;
return false;
}
Initialize the texture and blur shader for the blur object to use.
// Create and initialize the texture shader object.
m_TextureShader = new TextureShaderClass;
result = m_TextureShader->Initialize(m_OpenGL);
if(!result)
{
cout << "Error: Could not initialize the texture shader object." << endl;
return false;
}
// Create and initialize the blur shader object.
m_BlurShader = new BlurShaderClass;
result = m_BlurShader->Initialize(m_OpenGL);
if(!result)
{
cout << "Error: Could not initialize the blur shader object." << endl;
return false;
}
// Set the shadow map bias to fix the floating point precision issues (shadow acne/lines artifacts).
m_shadowMapBias = 0.0022f;
return true;
}
Release the newly created objects in the Shutdown function.
void ApplicationClass::Shutdown()
{
// Release the blur shader object.
if(m_BlurShader)
{
m_BlurShader->Shutdown();
delete m_BlurShader;
m_BlurShader = 0;
}
// Release the texture shader object.
if(m_TextureShader)
{
m_TextureShader->Shutdown();
delete m_TextureShader;
m_TextureShader = 0;
}
// Release the blur object.
if(m_Blur)
{
m_Blur->Shutdown();
delete m_Blur;
m_Blur = 0;
}
// Release the soft shadow shader object.
if(m_SoftShadowShader)
{
m_SoftShadowShader->Shutdown();
delete m_SoftShadowShader;
m_SoftShadowShader = 0;
}
// Release the shadow shader object.
if(m_ShadowShader)
{
m_ShadowShader->Shutdown();
delete m_ShadowShader;
m_ShadowShader = 0;
}
// Release the depth shader object.
if(m_DepthShader)
{
m_DepthShader->Shutdown();
delete m_DepthShader;
m_DepthShader = 0;
}
// Release the black and white render texture object.
if(m_BlackWhiteRenderTexture)
{
m_BlackWhiteRenderTexture->Shutdown();
delete m_BlackWhiteRenderTexture;
m_BlackWhiteRenderTexture = 0;
}
// Release the render texture object.
if(m_RenderTexture)
{
m_RenderTexture->Shutdown();
delete m_RenderTexture;
m_RenderTexture = 0;
}
// Release the light object.
if(m_Light)
{
delete m_Light;
m_Light = 0;
}
// Release the ground model object.
if(m_GroundModel)
{
m_GroundModel->Shutdown();
delete m_GroundModel;
m_GroundModel = 0;
}
// Release the sphere model object.
if(m_SphereModel)
{
m_SphereModel->Shutdown();
delete m_SphereModel;
m_SphereModel = 0;
}
// Release the cube model object.
if(m_CubeModel)
{
m_CubeModel->Shutdown();
delete m_CubeModel;
m_CubeModel = 0;
}
// Release the camera object.
if(m_Camera)
{
delete m_Camera;
m_Camera = 0;
}
// Release the OpenGL object.
if(m_OpenGL)
{
m_OpenGL->Shutdown();
delete m_OpenGL;
m_OpenGL = 0;
}
return;
}
bool ApplicationClass::Frame(InputClass* Input)
{
static float lightPositionX = -5.0f;
bool result;
// Check if the escape key has been pressed, if so quit.
if(Input->IsEscapePressed() == true)
{
return false;
}
// Update the position of the light each frame.
lightPositionX += 0.05f;
if(lightPositionX > 5.0f)
{
lightPositionX = -5.0f;
}
// Update the position of the light.
m_Light->SetPosition(lightPositionX, 8.0f, -5.0f);
m_Light->GenerateViewMatrix();
// Render the scene depth for the first light to it's render texture.
result = RenderDepthToTexture();
if(!result)
{
return false;
}
We have added a new step in the Frame function to render the shadowed scene in black and white to a render texture.
// Next render the shadowed scene in black and white.
result = RenderBlackAndWhiteShadows();
if(!result)
{
return false;
}
Once we have our black and white render texture of the scene with shadows, we can then blur it using the blur object.
// Blur the black and white shadow render texture using the BlurClass object.
result = m_Blur->BlurTexture(m_BlackWhiteRenderTexture, m_OpenGL, m_Camera, m_TextureShader, m_BlurShader);
if(!result)
{
return true;
}
// Render the final graphics scene.
result = Render();
if(!result)
{
return false;
}
return true;
}
bool ApplicationClass::RenderDepthToTexture()
{
float translateMatrix[16], lightViewMatrix[16], lightProjectionMatrix[16];
bool result;
// Set the render target to be the render texture and clear it.
m_RenderTexture->SetRenderTarget();
m_RenderTexture->ClearRenderTarget(0.0f, 0.0f, 0.0f, 1.0f);
// Get the view and projection matrices from the light object.
m_Light->GetViewMatrix(lightViewMatrix);
m_Light->GetProjectionMatrix(lightProjectionMatrix);
// Setup the translation matrix for the cube model.
m_OpenGL->MatrixTranslation(translateMatrix, -2.0f, 2.0f, 0.0f);
// Render the cube model using the depth shader and the light matrices.
result = m_DepthShader->SetShaderParameters(translateMatrix, lightViewMatrix, lightProjectionMatrix);
if(!result)
{
return false;
}
m_CubeModel->Render();
// Setup the translation matrix for the sphere model.
m_OpenGL->MatrixTranslation(translateMatrix, 2.0f, 2.0f, 0.0f);
// Render the sphere model using the depth shader and the light matrices.
result = m_DepthShader->SetShaderParameters(translateMatrix, lightViewMatrix, lightProjectionMatrix);
if(!result)
{
return false;
}
m_SphereModel->Render();
// Setup the translation matrix for the ground model.
m_OpenGL->MatrixTranslation(translateMatrix, 0.0f, 1.0f, 0.0f);
// Render the ground model using the depth shader and the light matrices.
result = m_DepthShader->SetShaderParameters(translateMatrix, lightViewMatrix, lightProjectionMatrix);
if(!result)
{
return false;
}
m_GroundModel->Render();
// Reset the render target back to the original back buffer and not the render to texture anymore. And reset the viewport back to the original.
m_OpenGL->SetBackBufferRenderTarget();
m_OpenGL->ResetViewport();
return true;
}
This new function is where we render the shadows as a black and white only image to a render texture.
It uses the modified shadow shader to do the work.
bool ApplicationClass::RenderBlackAndWhiteShadows()
{
float viewMatrix[16], projectionMatrix[16], lightViewMatrix[16], lightProjectionMatrix[16], translateMatrix[16];
float lightPosition[3];
bool result;
// Set the render target to be the black and white render texture and clear it.
m_BlackWhiteRenderTexture->SetRenderTarget();
m_BlackWhiteRenderTexture->ClearRenderTarget(0.0f, 0.0f, 0.0f, 1.0f);
// Get the view matrix from the camera, and get the projection matrix from the OpenGL object.
m_Camera->GetViewMatrix(viewMatrix);
m_OpenGL->GetProjectionMatrix(projectionMatrix);
// Get the view and projection matrices from the light object.
m_Light->GetViewMatrix(lightViewMatrix);
m_Light->GetProjectionMatrix(lightProjectionMatrix);
// Get the light position.
m_Light->GetPosition(lightPosition);
// Setup the translation matrix for the cube model.
m_OpenGL->MatrixTranslation(translateMatrix, -2.0f, 2.0f, 0.0f);
// Render the cube model using the shadow shader.
result = m_ShadowShader->SetShaderParameters(translateMatrix, viewMatrix, projectionMatrix, lightViewMatrix, lightProjectionMatrix, lightPosition, m_shadowMapBias);
if(!result)
{
return false;
}
// Set the render texture in texture unit 0.
m_RenderTexture->SetTexture(0);
m_CubeModel->Render();
// Setup the translation matrix for the sphere model.
m_OpenGL->MatrixTranslation(translateMatrix, 2.0f, 2.0f, 0.0f);
// Render the sphere model using the shadow shader.
result = m_ShadowShader->SetShaderParameters(translateMatrix, viewMatrix, projectionMatrix, lightViewMatrix, lightProjectionMatrix, lightPosition, m_shadowMapBias);
if(!result)
{
return false;
}
// Set the render texture in texture unit 0.
m_RenderTexture->SetTexture(0);
m_SphereModel->Render();
// Setup the translation matrix for the ground model.
m_OpenGL->MatrixTranslation(translateMatrix, 0.0f, 1.0f, 0.0f);
// Render the ground model using the shadow shader.
result = m_ShadowShader->SetShaderParameters(translateMatrix, viewMatrix, projectionMatrix, lightViewMatrix, lightProjectionMatrix, lightPosition, m_shadowMapBias);
if(!result)
{
return false;
}
// Set the render texture in texture unit 0.
m_RenderTexture->SetTexture(0);
m_GroundModel->Render();
// Reset the render target back to the original back buffer and not the render to texture anymore. And reset the viewport back to the original.
m_OpenGL->SetBackBufferRenderTarget();
m_OpenGL->ResetViewport();
return true;
}
bool ApplicationClass::Render()
{
float worldMatrix[16], viewMatrix[16], projectionMatrix[16];
float diffuseColor[4], ambientColor[4], lightPosition[3];
bool result;
// Clear the buffers to begin the scene.
m_OpenGL->BeginScene(0.0f, 0.0f, 0.0f, 1.0f);
// Get the world, view, and projection matrices from the opengl and camera objects.
m_OpenGL->GetWorldMatrix(worldMatrix);
m_Camera->GetViewMatrix(viewMatrix);
m_OpenGL->GetProjectionMatrix(projectionMatrix);
// Get the light properties.
m_Light->GetPosition(lightPosition);
m_Light->GetAmbientLight(ambientColor);
m_Light->GetDiffuseColor(diffuseColor);
// Setup the translation matrix for the cube model.
m_OpenGL->MatrixTranslation(worldMatrix, -2.0f, 2.0f, 0.0f);
Now when we render each of our objects, we use the soft shadow shader and give it the black and white blurred shadow texture for projecting our soft shadows onto the scene.
// Set the soft shadow shader as the current shader program and set the parameters that it will use for rendering.
result = m_SoftShadowShader->SetShaderParameters(worldMatrix, viewMatrix, projectionMatrix, lightPosition, ambientColor, diffuseColor);
if(!result)
{
return false;
}
// Set the blurred black and white shadow texture in texture unit 1.
m_BlackWhiteRenderTexture->SetTexture(1);
// Render the cube model using the soft shadow shader.
m_CubeModel->SetTexture1(0);
m_CubeModel->Render();
// Setup the translation matrix for the sphere model.
m_OpenGL->MatrixTranslation(worldMatrix, 2.0f, 2.0f, 0.0f);
// Set the shadow shader as the current shader program and set the parameters that it will use for rendering.
result = m_SoftShadowShader->SetShaderParameters(worldMatrix, viewMatrix, projectionMatrix, lightPosition, ambientColor, diffuseColor);
if(!result)
{
return false;
}
// Set the blurred black and white shadow texture in texture unit 1.
m_BlackWhiteRenderTexture->SetTexture(1);
// Render the sphere model using the soft shadow shader.
m_SphereModel->SetTexture1(0);
m_SphereModel->Render();
// Setup the translation matrix for the ground model.
m_OpenGL->MatrixTranslation(worldMatrix, 0.0f, 1.0f, 0.0f);
// Set the shadow shader as the current shader program and set the parameters that it will use for rendering.
result = m_SoftShadowShader->SetShaderParameters(worldMatrix, viewMatrix, projectionMatrix, lightPosition, ambientColor, diffuseColor);
if(!result)
{
return false;
}
// Set the blurred black and white shadow texture in texture unit 1.
m_BlackWhiteRenderTexture->SetTexture(1);
// Render the ground model using the soft shadow shader.
m_GroundModel->SetTexture1(0);
m_GroundModel->Render();
// Present the rendered scene to the screen.
m_OpenGL->EndScene();
return true;
}
Summary
The shadows now have soft edges creating a more realistic shadowing effect.
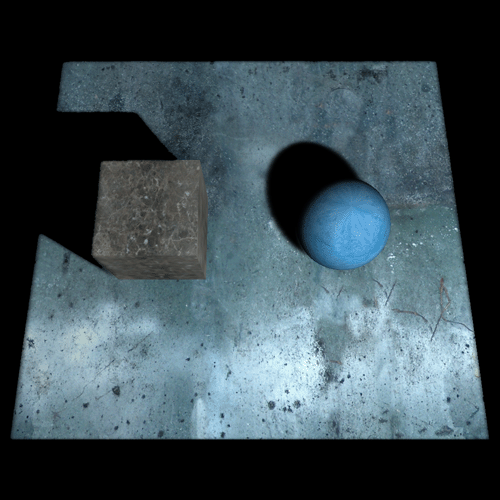
To Do Exercises
1. Recompile and run the program.
2. Change the blur effect to a faster performing blur. There are quite a few optimized techniques that can be found on the net.
Source Code
Source Code and Data Files: gl4linuxtut44_src.tar.gz